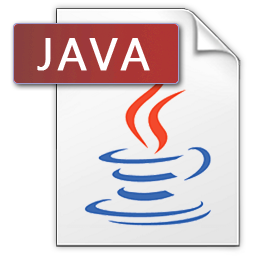
JFileChooser object allow you create Open File Dialog or Save File Dialog in Java. It's really powerful. Today, I will show you how to do that. I will create a swing application project for demonstration
public void mouseClicked(MouseEvent e) { JFileChooser fc = new JFileChooser(); int rv = fc.showOpenDialog(null); if (rv == JFileChooser.APPROVE_OPTION) { File file = fc.getSelectedFile(); String filePath = file.getAbsolutePath(); } }
filePath is the file you want to process.
2/ SaveFileDialog
public void mouseClicked(MouseEvent e) { JFileChooser fileChooser = new JFileChooser(); FileFilter filter = new FileFilter() { @Override public String getDescription() { // TODO Auto-generated method stub return "XML File | *.xml"; //Sample for file description } @Override public boolean accept(File f) { // TODO Auto-generated method stub if(f.isDirectory()) { return true; // show directories } return f.getName().contains("xml"); // Just show XML file only. } }; fileChooser.setFileFilter(filter); fileChooser.setDialogTitle("Specify a file to save"); int userSelection = fileChooser.showSaveDialog(null); if (userSelection == JFileChooser.APPROVE_OPTION) { File fileToSave = fileChooser.getSelectedFile(); String filePath = fileToSave.getAbsolutePath(); } }
No comments:
Post a Comment