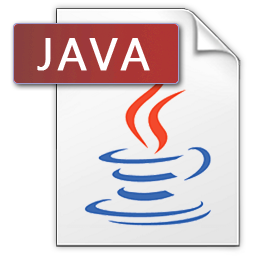
This post will show you how to send an email in Java application. I will use Gmail for demonstration. By default, Gmail supports you send email through smtp, but to be sure, there is gmail's setting information for your check
There is gmail setting for SMTP
props.put("mail.smtp.auth", "true"); props.put("mail.smtp.starttls.enable", "true"); props.put("mail.smtp.host", "smtp.gmail.com"); props.put("mail.smtp.port", "587"); // 587 for TSL required or 465 for SSL required
Now here we go
// Recipient's email ID needs to be mentioned. String to = "toEmail@gmail.com";//change accordingly // Sender's email ID needs to be mentioned String from = "fromEmail@gmail.com";//change accordingly final String username = "emailAccount";//change accordingly final String password = "********";//change accordingly // Assuming you are sending email through relay.jangosmtp.net String host = "smtp.gmail.com"; Properties props = new Properties(); props.put("mail.smtp.auth", "true"); props.put("mail.smtp.starttls.enable", "true"); props.put("mail.smtp.host", host); props.put("mail.smtp.port", "587"); // Get the Session object. Session session = Session.getInstance(props, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(username, password); } }); try { // Create a default MimeMessage object. Message message = new MimeMessage(session); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to)); // Set Subject: header field message.setSubject("Testing Subject"); // Now set the actual message message.setText("Hello, this is sample for to check send " + "email using JavaMailAPI "); // Send message Transport.send(message); System.out.println("Sent message successfully...."); } catch (MessagingException e) { throw new RuntimeException(e); }
These classes used above is imported from javax.mail package and it is not delivered to jre, so, you can download it from this link: mail.jar
No comments:
Post a Comment